- Joined
- Aug 2, 2016
- Posts
- 1,611
- Solutions
- 4
- Reaction
- 635
- Points
- 528
INTRODUCTION TO JAVA
Hello everyone,
This is a tutorial to teach you some of the basics of Java. I am not making this tutorial to share advanced java code or tutorials with you, this tutorial is designed for those specialists who have no (or very little) previous coding experience/knowledge, who would like to learn.
Introduction
Java is one of the most popular programming languages used today (particularly for client-server web applications). The developers of Java had 5 goals for the language: It should be "simple, object-oriented and familiar", "robust and secure", "architecture-neutral and portable", "high performance" and "interpreted, threaded, and dynamic". It has very similar syntax to C and C++, but it has fewer low-level facilities than either of them, because of the way java programs are run in the JVM. The advantage to the JVM is that it lets application developers "write once, run anywhere", because the code they write can be compiled to class files, inside a JAR (a Java Archive that contains a set of classes a program exists of). The JAR is runnable on any JVM without recompiling. It also has very few dependencies due to its portable design - everything you need comes with the java runtime which is installed on most computers. Disadvantages to the language is that it is not great for coding low level programs, and things such as malware, because it is not natively run and does not have the same abilities as a native language like c/c++ (unless you use the JNI), along with other factors.
Java is an object-oriented programming (OOP) language. OOP languages treat everything as an Object (although Java is not pure OOP - it has primitive types as well), and use abstraction, encapsulation, polymorphism, and inheritance. I will cover these things later.
space
Getting started
There are 3 different java releases: The Java Runtime Environment (JRE, which comes with the parts of the Java SE platform required to run Java programs and is intended for end-users), and the Java Development Kit (JDK, which is intended for software developers and includes development tools such as the Java compiler, Javadoc, Jar, and a debugger). There is also the server JRE. To start coding in Java, you must install the Java Development Kit.
Download the latest JDK 8 for your operating system here: You do not have permission to view the full content of this post. Log in or register now.
If you want to code using JDK 7, that can be found here: You do not have permission to view the full content of this post. Log in or register now.
After installing the JDK, you need to add an environment variable. Open "Control Panel > System > Advanced system settings > Environment Variables". Click New, call the variable Java, and link your JDK bin folder.
Next, you will need an IDE. An IDE is an Integrated Development Environment, combining the editor, compiler, management and error checking all into one easy environment.
You can code in Java without an IDE by using any text editor (notepad, notepad++, sublime text) and the command line compiler, but I think IDEs are better because they help prevent errors and they keep your project organised.
I use Eclipse (Download: You do not have permission to view the full content of this post. Log in or register now.), but other popular IDEs include NetBeans (Download: You do not have permission to view the full content of this post. Log in or register now.) and IntelliJ (Download: You do not have permission to view the full content of this post. Log in or register now.). Download and install an IDE.
IMPORTANT NOTE: Make sure all your versions match. If you have a 64 bit OS, make sure you download the 64 bit JDK and 64 bit eclipse.
Run eclipse and wait for it to load. Choose a workspace location to store your projects, and wait until the IDE itself loads.
To create a new Java project in eclipse, click "File > New > Java Project". Choose a name for your project and click "Finish". Now we can start coding.
Hello World
As usual, whenever we start a new programming language, the first program we always write is Hello World. In java, this is how you write a hello world program:
This is the breakdown of the project: We have a Class called HelloWorld which holds the main function of our program (main). When you run the program in eclipse (Run > Run), eclipse will find the main function (the main function is always called main) and run it. The function only has one line of code, which calls println("Hello World!"). All the println function does is print a line to the console. When you run this, you will see "Hello World!" written in the console. Your first java program!
If you want to compile and run the java program without using the IDE, then first run the compile command:
Then run the compiled program:
Classes
A class is the blueprint from which individual objects are created. Almost every object in Java is a class. Here is an example of a class:
Don't worry if you don't understand all that right now, we will be coming back to the Car class throughout this tutorial. Just notice now that the class contains 2 types of things: variables (mileage, manufacturer, petrol) and methods (refillPetrol, getMileage). There is also a Constructor method (Car) which we will cover shortly.
Classes are essential to OOP. Here are some of the things classes can do:
* Classes can be "instantiated", which means creating a new object based on that class. For example, if we have a Car class, we can create a new Car based on that class.
Instantiating new classes is very easy:
We just created a new Car object called myNewCar, and stored a new Car object in it using the "new" keyword.
* Classes can be "extended", also known as inheritance. This allows us to make "subclasses" of a template class, which all share similar attributes/functionality, to save us from repeating ourselves.
Classes are extended using the "extends" keyword - what a suprise. For example, we can create a child class of Car called ToyotaCar, because it shares very similar attributes such as remainingPetrol, and functions such as turnLeft():
Once again, don't worry about the ToyotaCar constructor yet. We will cover that shortly. If we want to make the class ONLY extendable, so it cannot be used (instantiated) on its own, we use the "abstract" keyword in its declaration. Abstract methods are methods which are given no body, but which HAVE to be included in subclasses with a body. This can allow us to create a "template" class and all the information must be filled in later.
Example of an abstract method:
The abstract method has no body, just a name, to make sure that classes which inherit this class include the method. When inheriting that class, we would write:
You can also override a normal method in a subclass too, even if it isnt abstract, in case you want to change something in it. An overriden method can call the superclass method using the super keyword:
Variables
A variable is a container that holds values. Each variable is declared to be a certain type - depending on whether it holds text, integers, true or false, etc.
There are eight primitive data types:
* byte - Values from -128 to 127 (inclusive).
* short - values from -32,768 to 32,767 (inclusive).
* int - this is the standard variable uses to store whole numbers. it stores a huge range of positive and negative.
* long - double the memory size of an integer - can hold much larger numbers.
* float - Used instead of double to conserve memory, with less accuracy.
* double - standard type to use for decimal numbers.
* char - a single 16-bit Unicode character. ranges from '\u0000' to '\uffff'. An array of chars (a CharSequence) is used to store text - a string is a subclass of CharSequence so a string is very similar to an array of chars.
* boolean - only 2 possible values: true or false.
Types like byte and short are used to save memory, because they are much smaller than the larger int, but they cannot hold as large a range.
In the car class, we can see there are 3 variables declared: mileage, manufacturer and petrol. Mileage and Petrol are both doubles, because they store decimal numbers. manufacturer is a String, which stores text.
Declaration:
This will declare a variable of type [type]. name can be any name, except for keywords already used by Java (such as string). But this new variable has no value - it is an empty container.
You can also declare multiple variables of the same type at once:
Or, assigning a value at the same time:
Initialization / Assignment:
This stores [value] in the variable. The value must be the same type as name, otherwise you must "typecast". You can assign the value in the same line as the declaration too. You must assign the variable a value to use it.
Numerical variables can be used in many mathematical operations, like addition, multiplication, etc. Strings can also be manipulated, for example, concatenating strings together to form a longer string. In the code above, I demonstrated a slightly special operator - "petrol += amount;"
This += adds amount to the currently existing value of petrol. It is identical, but shorter, to writing "petrol = petrol + amount;"
You can read about the other operators in the Operators section.
space
Functions and Methods
Methods are blocks of code that can actually be run and do things. An example of a method from our car class is this:
Notice that we used the public modifier on this method, so that it can be accessed and run from outside the car class itself - for example, if we wanted to create a new car and refill it with some petrol in our main method.
The main() method is the method that is run when the program starts. It usually looks like this:
Lets have a look at how a function is coded:
A method is called like this:
Easy, right? If you want to run a method which is inside an instantiated class (or a static method), we can do it like this:
Modifiers
In the code, you will notice these 3 variables declared:
Now, you might be wondering what these random private and public words are. they are known as modifiers, and control the behaviour of the variabl/objecte you are declaring. Here are some common modifiers:
- public. This modifier allows the variable to be accessed directly from other classes, the value to be read and modifier, etc. This is often discouraged because the class then has no control over what changes are made, so we often use "getter" and "setter" methods to change the variables with checks on what they are being changed to.
- private. As you might have guessed, this is the opposite of public. This modifier allows only the code in the class to read and change the variable. Things outside the class do not know the variable exists, and cannot access it, so we must create getter and setter methods to read and change the value of private variables. For example, mileage is a private variable, so I created a "getMileage()" method to read the value from outside the class.
- protected. This is somewhere in the middle of private and public. This lets only the class and its children classes access the variable, but not other classes.
Tip: It is good practice to use the use the most restrictive access level that makes sense for a particular member. Use private unless you have a good reason not to.
Access levels
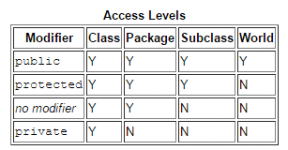
- static. This modifier on an object means that only ONE copy of that object is created in the program. If we created a static variable in the car class, then no matter how many cars we instantiated, all the instances of that variable would actually have the same value, and changing one would change the others, because they are all the same. Static methods cannot access non-static methods.
- abstract. This is used in a class of method declaration to force that class to be inherited, before it can be instantiated, for example if the method needed to be changed and could not be used on its own (like if we created an animal class, we cannot create a new animal, we would instead have to extend it to make a cat and then create a new cat)
- final. This is used to make something unchangeable. A final variable is a constant, a final method cannot be overridden and a final class cannot be subclassed.
There are a few other modifiers, but these are probably the most common. I will explain the others if we come across them later. Modifiers are keywords in Java, so they cannot be used as variable names - they are set aside by Java for their specific purpose. Other keywords can be found here: You do not have permission to view the full content of this post. Log in or register now.
Hello everyone,
This is a tutorial to teach you some of the basics of Java. I am not making this tutorial to share advanced java code or tutorials with you, this tutorial is designed for those specialists who have no (or very little) previous coding experience/knowledge, who would like to learn.
Introduction
Java is one of the most popular programming languages used today (particularly for client-server web applications). The developers of Java had 5 goals for the language: It should be "simple, object-oriented and familiar", "robust and secure", "architecture-neutral and portable", "high performance" and "interpreted, threaded, and dynamic". It has very similar syntax to C and C++, but it has fewer low-level facilities than either of them, because of the way java programs are run in the JVM. The advantage to the JVM is that it lets application developers "write once, run anywhere", because the code they write can be compiled to class files, inside a JAR (a Java Archive that contains a set of classes a program exists of). The JAR is runnable on any JVM without recompiling. It also has very few dependencies due to its portable design - everything you need comes with the java runtime which is installed on most computers. Disadvantages to the language is that it is not great for coding low level programs, and things such as malware, because it is not natively run and does not have the same abilities as a native language like c/c++ (unless you use the JNI), along with other factors.
Java is an object-oriented programming (OOP) language. OOP languages treat everything as an Object (although Java is not pure OOP - it has primitive types as well), and use abstraction, encapsulation, polymorphism, and inheritance. I will cover these things later.
space
Getting started
There are 3 different java releases: The Java Runtime Environment (JRE, which comes with the parts of the Java SE platform required to run Java programs and is intended for end-users), and the Java Development Kit (JDK, which is intended for software developers and includes development tools such as the Java compiler, Javadoc, Jar, and a debugger). There is also the server JRE. To start coding in Java, you must install the Java Development Kit.
Download the latest JDK 8 for your operating system here: You do not have permission to view the full content of this post. Log in or register now.
If you want to code using JDK 7, that can be found here: You do not have permission to view the full content of this post. Log in or register now.
After installing the JDK, you need to add an environment variable. Open "Control Panel > System > Advanced system settings > Environment Variables". Click New, call the variable Java, and link your JDK bin folder.
Next, you will need an IDE. An IDE is an Integrated Development Environment, combining the editor, compiler, management and error checking all into one easy environment.
You can code in Java without an IDE by using any text editor (notepad, notepad++, sublime text) and the command line compiler, but I think IDEs are better because they help prevent errors and they keep your project organised.
I use Eclipse (Download: You do not have permission to view the full content of this post. Log in or register now.), but other popular IDEs include NetBeans (Download: You do not have permission to view the full content of this post. Log in or register now.) and IntelliJ (Download: You do not have permission to view the full content of this post. Log in or register now.). Download and install an IDE.
IMPORTANT NOTE: Make sure all your versions match. If you have a 64 bit OS, make sure you download the 64 bit JDK and 64 bit eclipse.
Run eclipse and wait for it to load. Choose a workspace location to store your projects, and wait until the IDE itself loads.
To create a new Java project in eclipse, click "File > New > Java Project". Choose a name for your project and click "Finish". Now we can start coding.
Hello World
As usual, whenever we start a new programming language, the first program we always write is Hello World. In java, this is how you write a hello world program:
Code:
class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello World!");
}
}
This is the breakdown of the project: We have a Class called HelloWorld which holds the main function of our program (main). When you run the program in eclipse (Run > Run), eclipse will find the main function (the main function is always called main) and run it. The function only has one line of code, which calls println("Hello World!"). All the println function does is print a line to the console. When you run this, you will see "Hello World!" written in the console. Your first java program!
If you want to compile and run the java program without using the IDE, then first run the compile command:
Code:
javac HelloWorld.java
Then run the compiled program:
Code:
java HelloWorld
Classes
A class is the blueprint from which individual objects are created. Almost every object in Java is a class. Here is an example of a class:
Code:
public class Car
{
private double mileage;
public String manufacturer = "";
private double petrol;
public Car(String manufacturer)
{
this.manufacturer = manufacturer;
mileage = 0;
petrol = 0;
}
public void refillPetrol(double amount)
{
petrol += amount;
}
public double getMileage()
{
return mileage;
}
}
Don't worry if you don't understand all that right now, we will be coming back to the Car class throughout this tutorial. Just notice now that the class contains 2 types of things: variables (mileage, manufacturer, petrol) and methods (refillPetrol, getMileage). There is also a Constructor method (Car) which we will cover shortly.
Classes are essential to OOP. Here are some of the things classes can do:
* Classes can be "instantiated", which means creating a new object based on that class. For example, if we have a Car class, we can create a new Car based on that class.
Instantiating new classes is very easy:
Code:
Car myNewCar = new Car();
We just created a new Car object called myNewCar, and stored a new Car object in it using the "new" keyword.
* Classes can be "extended", also known as inheritance. This allows us to make "subclasses" of a template class, which all share similar attributes/functionality, to save us from repeating ourselves.
Classes are extended using the "extends" keyword - what a suprise. For example, we can create a child class of Car called ToyotaCar, because it shares very similar attributes such as remainingPetrol, and functions such as turnLeft():
Code:
public class ToyotaCar extends Car
{
public ToyotaCar()
{
super("Toyota");
}
}
Once again, don't worry about the ToyotaCar constructor yet. We will cover that shortly. If we want to make the class ONLY extendable, so it cannot be used (instantiated) on its own, we use the "abstract" keyword in its declaration. Abstract methods are methods which are given no body, but which HAVE to be included in subclasses with a body. This can allow us to create a "template" class and all the information must be filled in later.
Example of an abstract method:
Code:
abstract void turnLeft();
The abstract method has no body, just a name, to make sure that classes which inherit this class include the method. When inheriting that class, we would write:
Code:
public void turnLeft()
{
//code goes here
}
You can also override a normal method in a subclass too, even if it isnt abstract, in case you want to change something in it. An overriden method can call the superclass method using the super keyword:
Code:
public void turnLeft()
{
//this is the overriden turnLeft method. to call the original method, we use:
super.turnLeft();
}
Variables
A variable is a container that holds values. Each variable is declared to be a certain type - depending on whether it holds text, integers, true or false, etc.
There are eight primitive data types:
* byte - Values from -128 to 127 (inclusive).
* short - values from -32,768 to 32,767 (inclusive).
* int - this is the standard variable uses to store whole numbers. it stores a huge range of positive and negative.
* long - double the memory size of an integer - can hold much larger numbers.
* float - Used instead of double to conserve memory, with less accuracy.
* double - standard type to use for decimal numbers.
* char - a single 16-bit Unicode character. ranges from '\u0000' to '\uffff'. An array of chars (a CharSequence) is used to store text - a string is a subclass of CharSequence so a string is very similar to an array of chars.
* boolean - only 2 possible values: true or false.
Types like byte and short are used to save memory, because they are much smaller than the larger int, but they cannot hold as large a range.
In the car class, we can see there are 3 variables declared: mileage, manufacturer and petrol. Mileage and Petrol are both doubles, because they store decimal numbers. manufacturer is a String, which stores text.
Declaration:
Code:
[modifiers] [type] name;
This will declare a variable of type [type]. name can be any name, except for keywords already used by Java (such as string). But this new variable has no value - it is an empty container.
You can also declare multiple variables of the same type at once:
Code:
int a, b, c;
Code:
int a = 10, b = 3, c = -4;
Initialization / Assignment:
Code:
name = [value];
This stores [value] in the variable. The value must be the same type as name, otherwise you must "typecast". You can assign the value in the same line as the declaration too. You must assign the variable a value to use it.
Numerical variables can be used in many mathematical operations, like addition, multiplication, etc. Strings can also be manipulated, for example, concatenating strings together to form a longer string. In the code above, I demonstrated a slightly special operator - "petrol += amount;"
This += adds amount to the currently existing value of petrol. It is identical, but shorter, to writing "petrol = petrol + amount;"
You can read about the other operators in the Operators section.
space
Functions and Methods
Methods are blocks of code that can actually be run and do things. An example of a method from our car class is this:
Code:
public void refillPetrol(double amount)
{
petrol = petrol + amount;
}
Notice that we used the public modifier on this method, so that it can be accessed and run from outside the car class itself - for example, if we wanted to create a new car and refill it with some petrol in our main method.
The main() method is the method that is run when the program starts. It usually looks like this:
Code:
public static void main(String [] args)
{
}
Lets have a look at how a function is coded:
- we first have modifiers. In the refill class, we just made it public, but in main, we made it public static.
- next we have a return type. This is the type of variable/object the function will return when it is finished. Both functions i gave above have a return type "void" which means they do not return a value. but the getMileage() method in the car class has return type "double" which means it returns a value of type double to whatever called the method.
- then we have the function name. main is the name given to the main class, but you can give any other functions any name you like as long as it is not the same as a java keyword (like static or double).
- we have some parentheses. These can be empty or can have a variable declaration in them. The variables inside the parentheses are "parameters", which are values passed to the function so that it can do something with them. if the brackets are empty, then the function doesn't take any arguments.
- curly braces are used to surround the function body, where all the code that the function will do is put.
- finally, we need a return statement if the function has a return type (other than void). For example, if our function is returning a double, we must write return 12.0; at the end.
A method is called like this:
Code:
somewhereToStoreTheReturnedValue = methodname("arguement value");
Easy, right? If you want to run a method which is inside an instantiated class (or a static method), we can do it like this:
Code:
Car myNewCar = new Car();
myNewCar.refillPetrol(18.0);
double mileage = myNewCar.getMileage();
Modifiers
In the code, you will notice these 3 variables declared:
Code:
private float mileage;
public String manufacturer = "";
private float petrol;
Now, you might be wondering what these random private and public words are. they are known as modifiers, and control the behaviour of the variabl/objecte you are declaring. Here are some common modifiers:
- public. This modifier allows the variable to be accessed directly from other classes, the value to be read and modifier, etc. This is often discouraged because the class then has no control over what changes are made, so we often use "getter" and "setter" methods to change the variables with checks on what they are being changed to.
- private. As you might have guessed, this is the opposite of public. This modifier allows only the code in the class to read and change the variable. Things outside the class do not know the variable exists, and cannot access it, so we must create getter and setter methods to read and change the value of private variables. For example, mileage is a private variable, so I created a "getMileage()" method to read the value from outside the class.
- protected. This is somewhere in the middle of private and public. This lets only the class and its children classes access the variable, but not other classes.
Tip: It is good practice to use the use the most restrictive access level that makes sense for a particular member. Use private unless you have a good reason not to.
Access levels
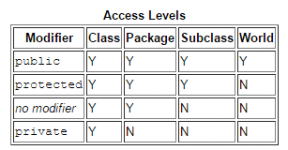
- static. This modifier on an object means that only ONE copy of that object is created in the program. If we created a static variable in the car class, then no matter how many cars we instantiated, all the instances of that variable would actually have the same value, and changing one would change the others, because they are all the same. Static methods cannot access non-static methods.
- abstract. This is used in a class of method declaration to force that class to be inherited, before it can be instantiated, for example if the method needed to be changed and could not be used on its own (like if we created an animal class, we cannot create a new animal, we would instead have to extend it to make a cat and then create a new cat)
- final. This is used to make something unchangeable. A final variable is a constant, a final method cannot be overridden and a final class cannot be subclassed.
There are a few other modifiers, but these are probably the most common. I will explain the others if we come across them later. Modifiers are keywords in Java, so they cannot be used as variable names - they are set aside by Java for their specific purpose. Other keywords can be found here: You do not have permission to view the full content of this post. Log in or register now.
Attachments
-
You do not have permission to view the full content of this post. Log in or register now.